Gift subscriptions are a fantastic feature that WooCommerce store owners can offer to their customers. However, handling gifted subscriptions requires unique processes, especially when it comes to managing subscription renewals.
Today, I’ll show you how to automatically set gifted WooCommerce subscriptions to manual renewal while leaving regular subscriptions untouched. This ensures your gifting experience is smooth and hassle-free for recipients and givers alike.
Why Set Gifted Subscriptions to Manual Renewal?
By default, WooCommerce Subscriptions automatically renew payments unless configured otherwise. For gifted subscriptions, automatic renewals may not be ideal because:
- The subscription giver might not want to be charged repeatedly without opting in.
- The recipient has no control over the renewal process, which could create confusion.
- Managing renewals manually allows the giver to decide whether to continue the gift for another cycle.
To address these scenarios, you can implement a simple solution to handle gifted subscriptions differently.
The Solution: Dynamic Code Implementation
Using the WooCommerce Subscriptions Gifting plugin, I’ve created a code snippet that dynamically detects gifted subscriptions. It automatically sets them to manual renewal and cancels any scheduled renewal actions.
// Hook into the subscription creation process
add_action( 'woocommerce_checkout_subscription_created', 'set_gifted_subscription_to_manual_renewal', 10, 2 );
function set_gifted_subscription_to_manual_renewal( $subscription, $order ) {
// Check if the subscription is gifted using the gifting plugin's functionality
if ( class_exists( 'WCS_Gifting' ) && WCS_Gifting::is_gifted_subscription( $subscription ) ) {
// Set the subscription to manual renewal
$subscription->set_requires_manual_renewal( true );
// Cancel any scheduled renewal actions
as_unschedule_all_actions( 'woocommerce_scheduled_subscription_payment', [ 'subscription_id' => $subscription->get_id() ] );
// Log the action for debugging
wc_get_logger()->info(
'Gifted subscription ID ' . $subscription->get_id() . ' renewals canceled and set to manual.',
[ 'source' => 'woocommerce' ]
);
// Save the subscription changes
$subscription->save();
}
}
// Additional safeguard: Ensure gifted subscriptions remain manual during payment
add_action( 'woocommerce_subscription_payment_complete', 'cancel_gifted_subscription_renewals', 10, 1 );
function cancel_gifted_subscription_renewals( $subscription ) {
// Verify gifted subscription using the gifting plugin
if ( class_exists( 'WCS_Gifting' ) && WCS_Gifting::is_gifted_subscription( $subscription ) ) {
// Cancel scheduled renewal actions
as_unschedule_all_actions( 'woocommerce_scheduled_subscription_payment', [ 'subscription_id' => $subscription->get_id() ] );
// Log the cancellation for confirmation
wc_get_logger()->info(
'Gifted subscription ID ' . $subscription->get_id() . ' scheduled renewals canceled post-payment.',
[ 'source' => 'woocommerce' ]
);
}
}
You can check out the code here too: https://gist.github.com/shameemreza/c4797e4be0118a412d4f0479bab958d7
Step-by-Step Implementation
- Add the Code: Copy the code snippet and paste it into your child theme’s
functions.php
file or a custom plugin. - Test Your Configuration: Place a test order for both regular and gifted subscriptions. Verify the behavior:
- Gifted Subscriptions: Should show “Via Manual Renewal.”
- Regular Subscriptions: Should retain their automatic renewal status.
- Verify the Logs: Check WooCommerce logs for confirmation messages about canceled renewals for gifted subscriptions.
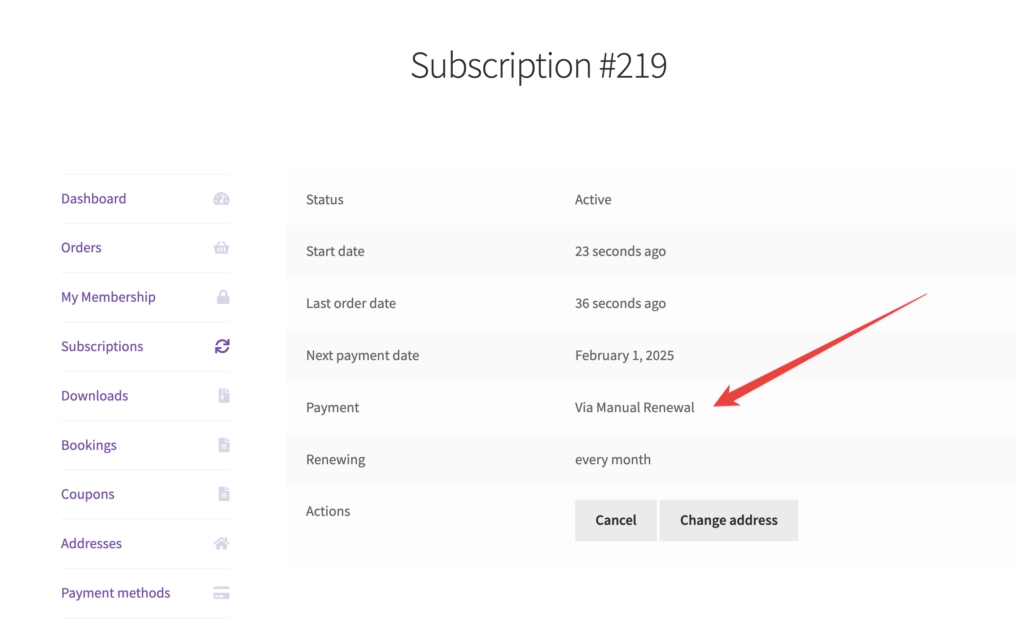
How It Works
- Gifted Subscription Detection: The code leverages the WooCommerce Subscriptions Gifting plugin’s API to check if a subscription is gifted.
- Automated Manual Renewal Configuration: When a gifted subscription is created, the code:
- Sets it to manual renewal.
- Cancels any scheduled renewal actions.
- Seamless Regular Subscription Processing: Non-gifted subscriptions are unaffected, ensuring they continue with automatic renewals as expected.
Benefits of This Approach
- Customer Satisfaction: Recipients and givers have clarity on the subscription lifecycle.
- Automation: The process requires no manual intervention, saving time and reducing errors.
- Flexibility: Works seamlessly for all subscription product types (simple, variable, downloadable, virtual).
- Future-proof: The dynamic detection logic ensures compatibility with various subscription setups.
Frequently Asked Questions
Q: Will this affect regular subscriptions?
A: No, the code ensures only gifted subscriptions are modified. Regular subscriptions will continue with automatic renewal.
Q: Does this require the WooCommerce Subscriptions Gifting plugin?
A: Yes, this solution relies on the gifting plugin to identify gifted subscriptions.
Q: Can I apply this to existing gifted subscriptions?
A: Absolutely. You can extend this code to scan and update existing gifted subscriptions.
Conclusion
Managing gifted subscriptions in WooCommerce doesn’t have to be a hassle. With this simple implementation, you can ensure gifted subscriptions are handled with care, giving both the givers and recipients the flexibility they need.
Got questions or need help with WooCommerce customization? Drop them in the comments below!
Leave a Reply