You’re trying to load your WooCommerce product page or use a theme feature, and suddenly, nothing works. You check the browser console and there it is:
Uncaught ReferenceError: jQuery is not defined
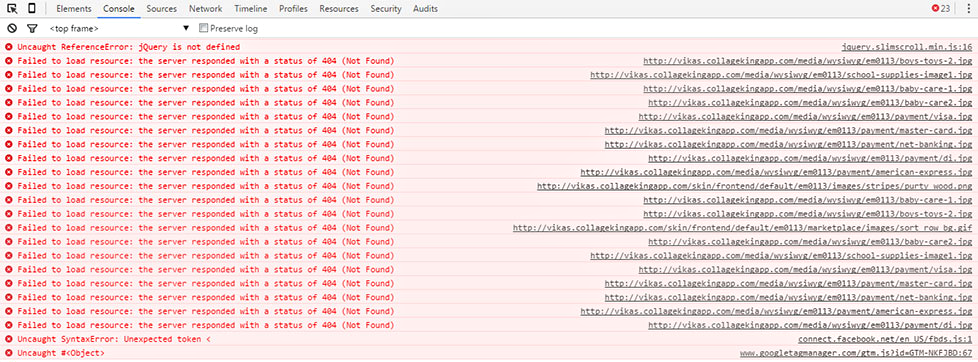
It’s one of the most common (and confusing) JavaScript errors WordPress developers and site owners run into. But don’t worry, we’ll break down what it means, why it happens, and how to fix it step-by-step.
What Does “jQuery is Not Defined” Actually Mean?
In plain terms: something on your site tried to run jQuery before it was loaded.
WordPress relies on jQuery for many frontend interactions, especially with themes and plugins. When that script isn’t available yet, the browser throws this error and stops whatever script was trying to run.
Common Causes of the Error
- jQuery wasn’t properly enqueued
- Scripts are loading in the wrong order
- A plugin deregistered jQuery (or loaded a conflicting version)
- You’re using a CDN version that’s blocked or failed to load
- Your optimization plugin is delaying or deferring jQuery
Step-by-Step: How to Fix It
1. Make Sure jQuery Is Properly Enqueued
Add this snippet to your theme’s functions.php
file or through a plugin like Code Snippets:
function ensure_jquery_loaded() {
wp_enqueue_script('jquery');
}
add_action('wp_enqueue_scripts', 'ensure_jquery_loaded');
WordPress ships with jQuery, so there’s no need to include it manually from external sources unless you have a specific reason.
2. Disable JavaScript Optimization Temporarily
If you use WP Rocket, Autoptimize, or a similar performance plugin, try this:
- Go to the JavaScript optimization settings.
- Exclude jQuery from combining, deferring, or delaying.
For WP Rocket, add this to the “Excluded JavaScript file”:
/wp-includes/js/jquery/jquery.min.js
3. Use SCRIPT_DEBUG to Load Unminified Scripts
In your wp-config.php
, enable debug mode for scripts:
define('SCRIPT_DEBUG', true);
This forces WordPress to load the original script versions. It’s a great way to see if a minification or combination issue is at fault.
4. Check the Script Loading Order
Your custom script might be trying to use jQuery before it’s loaded. To fix this, ensure your script depends on jquery
in wp_enqueue_script()
:
wp_enqueue_script('my-custom-script', get_template_directory_uri() . '/js/custom.js', array('jquery'), null, true);
This makes sure jQuery loads before your script.
5. Look for CDN or External jQuery Versions
If your site uses a CDN-hosted jQuery (e.g. from Google), test if that URL is blocked or slow.
Fallback example:
<script src="//ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
window.jQuery || document.write('<script src="/js/jquery.min.js"><\/script>');
</script>
Using WordPress’s built-in version is still the safest choice in most cases.
6. Check for Plugin or Theme Conflicts
Disable plugins one by one to see if one of them is deregistering jQuery.
Also, test by switching to a default theme like Twenty Twenty-Five. If the error disappears, the problem is likely in your theme’s scripts.
Bonus: How to Spot the Error in the First Place
- Open your browser console (F12 or Cmd+Option+J).
- Reload your page.
- If the error is there, it’ll show up in red:
Uncaught ReferenceError: jQuery is not defined
If you’re contacting WooCommerce or WordPress support, a screenshot of this message helps a lot.
Still Seeing the Error?
Try this last-resort trick in wp-config.php
:
define('CONCATENATE_SCRIPTS', false);
It disables script concatenation, useful for debugging script load order problems.
Wrapping Up
“jQuery is not defined” is frustrating, but almost always fixable with the right approach:
- Load jQuery the right way
- Avoid conflicting script optimizations
- Use WordPress functions to manage dependencies
- Lean on debug tools like the browser console or
SCRIPT_DEBUG
Once resolved, your theme scripts, plugins, and frontend interactivity will start behaving again, and your customers will thank you for it.
Leave a Reply