Back when I first got into WordPress development, one of the trickiest bugs to deal with was… nothing.
Literally nothing.
You’d save some changes, refresh the page, and nothing looked different. Turns out, the culprit was often cached data – especially in block themes using theme.json
.
That’s where WordPress 6.3’s new WP_DEVELOPMENT_MODE
constant changes the game.
Let’s break down what this new constant does, how it helps, and how to set it up properly for your projects.
What is WP_DEVELOPMENT_MODE?
WordPress 6.3 introduced a new constant called WP_DEVELOPMENT_MODE
. It’s designed to tell WordPress what kind of development you’re doing – theme, plugin, core, or all – and adjusts how the system behaves behind the scenes.
If you’re building something for a client or contributing to the ecosystem, setting this constant can make your workflow smoother and your changes show up the way you expect.
Here are the valid values:
'theme'
: You’re developing a theme.'plugin'
: You’re building or testing a plugin.'core'
: You’re working on WordPress Core itself.'all'
: A catch-all when you’re doing a bit of everything.''
(empty string): The default when not set, used for live/production sites.
Why You Should Use It?
Right now, the main reason to set WP_DEVELOPMENT_MODE
is to disable theme.json
caching.
By default, WordPress caches theme.json
output to improve performance. That’s great for production, but a headache during development. With caching on, you might edit your theme’s JSON settings and not see the changes right away.
By setting WP_DEVELOPMENT_MODE
to 'theme'
(or 'all'
), WordPress skips that cache, so your updates appear immediately. No more guessing if your changes are working or stuck behind old data.
How to Set WordPress Development Mode?
You’ll need to add this to your wp-config.php
file. Make sure it’s before WordPress loads, so ideally, right after the database settings.
Example:
define( 'WP_DEVELOPMENT_MODE', 'theme' );
Or if you’re working on plugins:
define( 'WP_DEVELOPMENT_MODE', 'plugin' );
And if you’re doing it all:
define( 'WP_DEVELOPMENT_MODE', 'all' );
For shared environments where config values depend on the environment, you might do something like:
if ( defined( 'WP_ENVIRONMENT_TYPE' ) && WP_ENVIRONMENT_TYPE !== 'production' ) {
define( 'WP_DEVELOPMENT_MODE', 'all' );
}
Or using environment variables:
if ( function_exists( 'getenv' ) ) {
$env = getenv( 'WP_ENVIRONMENT_TYPE' );
if ( $env && $env !== 'production' ) {
define( 'WP_DEVELOPMENT_MODE', 'all' );
}
}
Quick Tip: How to Check If It’s Working?
In WordPress 6.3 and later, head to Tools → Site Health → Info → WordPress Constants. You’ll see the current value of WP_DEVELOPMENT_MODE
.
Or use this function in your code:
if ( wp_is_development_mode( 'theme' ) ) {
// Run theme-specific dev logic here
}
This function returns true
if the mode matches, or always true if set to 'all'
.
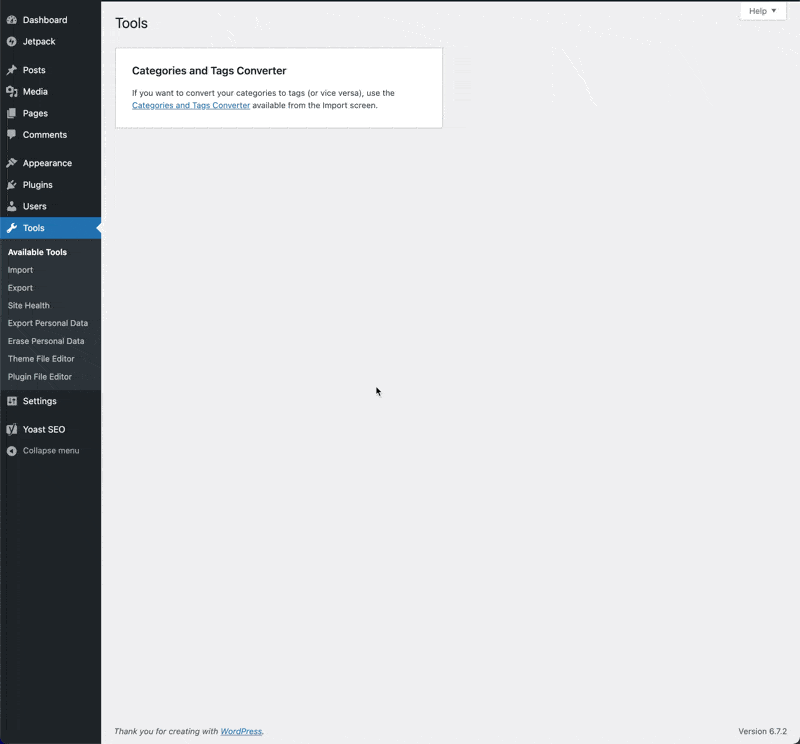
Common Mistakes to Avoid
- Defining it too late: If you put the constant after WordPress loads, it won’t work.
- Forgetting to disable it in production: Keep it off on live sites for better performance.
- Using the wrong scope: Only set
'core'
if you’re contributing to WordPress Core. For most real-world work,'plugin'
,'theme'
, or'all'
are better fits.
Final Thoughts
WP_DEVELOPMENT_MODE
won’t be the most visible part of your workflow, but it might be one of the most helpful. Especially with modern WordPress development relying so heavily on cached configurations.
So before you dive into building your next plugin or theme, set this constant. You’ll save yourself hours of confusion and give your development environment the clarity it needs.
Got questions or want to share your experience with using it? Let’s chat in the comments.
Reference:
- https://make.wordpress.org/core/2023/07/14/configuring-development-mode-in-6-3/
- https://developer.wordpress.org/reference/functions/wp_get_development_mode/
Leave a Reply